Developers
Validating Webhook Signatures
Introduction
When Giftogram sends a webhook to your application, it includes a signature in the
x-giftogram-signature
header. This signature allows you to verify the authenticity of the request and ensure that it indeed came from Giftogram.Starting from this release, a client secret can be associated with your API key, which you can use to perform cryptographic validation of incoming webhooks.
Obtaining Your Client Secret
- Navigate to Your API Keys
- From your Giftogram dashboard (or sandbox environment), click on the gear icon in the top-right corner to open Settings.
- Locate Your API Key
- In the API section (bottom-left), you’ll see your list of API keys.
- View Client Secret
- Each API key entry can now list your client secret. This secret is a unique token used exclusively for signature validation of webhooks.
Preview
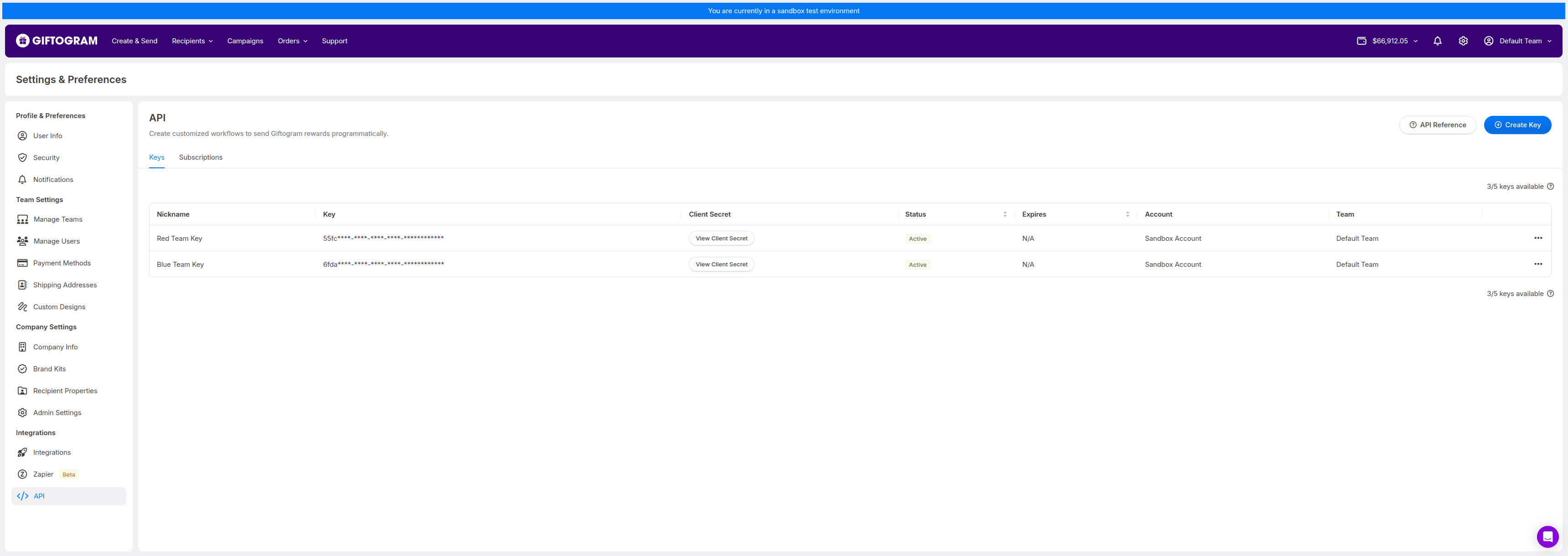
Preview
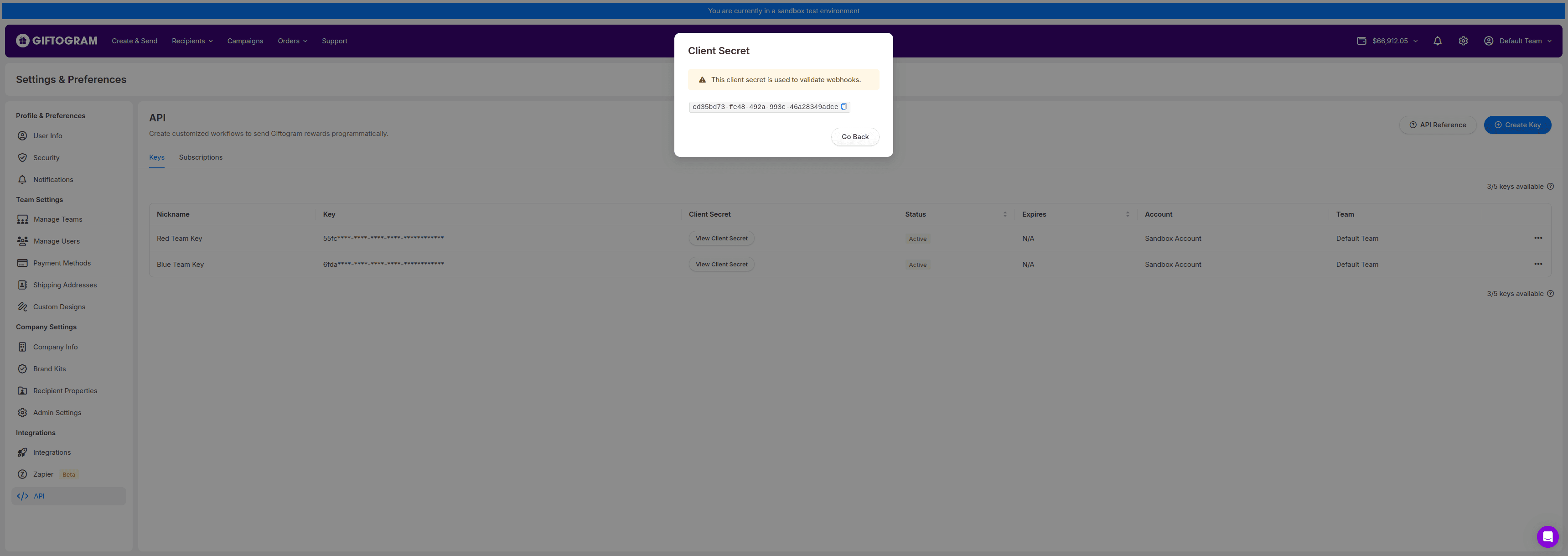
Keep your client secret as secure as you would your API key. It must not be shared or exposed in public code repositories.
Verifying Webhook Signatures
To verify an incoming webhook request:
- Retrieve the raw JSON payload from the request body (before you perform any transformations or modifications on it).
- Encode this raw payload as a JSON string (if it is not already in that format).
- Use your client secret to compute an HMAC-SHA256 hash of the encoded payload.
- Compare the resulting hash value to the
x-giftogram-signature
header. If they match, the webhook is validated.
Below is an example in PHP demonstrating how to generate and compare this signature.
<?php // Step 1: Obtain your client secret (from Giftogram's dashboard). // Note: Replace the placeholder with your actual client secret. Typically, this would be stored securely and accessed // from a configuration file or environment variable. $clientSecret = '12345678-9123-4567-8912-345678912345'; // Step 2: Capture the raw request payload from the webhook. // For example, using file_get_contents in PHP: $rawPayload = file_get_contents('php://input'); // Step 3: Convert the raw payload to a JSON-encoded string if needed. // In most frameworks, $rawPayload may already be a JSON string: $encodedPayload = json_encode(json_decode($rawPayload, true)); // Step 4: Compute the HMAC-SHA256 hash with the client secret. $computedSignature = hash_hmac('sha256', $encodedPayload, $clientSecret); // Step 5: Retrieve the provided signature from the webhook headers. // In this example, we assume it's passed as x-giftogram-signature. $providedSignature = $_SERVER['HTTP_X_GIFTOGRAM_SIGNATURE'] ?? ''; // Step 6: Compare the signatures to validate. if (hash_equals($computedSignature, $providedSignature)) { // The webhook is valid. http_response_code(200); echo 'Webhook signature validated successfully!'; } else { // The webhook signature does not match. http_response_code(400); echo 'Invalid signature.'; }